Implementing the SMARTER approach with Salient Vision® requires a combination of software configuration, data acquisition setup, and potentially custom code development. Here’s a detailed breakdown of the steps involved:
1. Set up Salient Vision® System:
- Install Salient Vision® Software: Install the Salient Vision® software on your system according to the provided instructions. Ensure you have the necessary licenses and access credentials.
- Configure Salient Sensors: Connect and configure the Salient Vision® sensors (cameras, etc.) to your system. This may involve setting up network connections, configuring camera parameters (resolution, frame rate, etc.), and calibrating the sensors for accurate data capture.
- Define Regions of Interest (ROIs): Within the Salient Vision® software, define the specific regions of interest (ROIs) within the camera’s field of view that you want to analyze. This helps focus the analysis on the most relevant areas.
2. Implement Data Acquisition (acquire_data
)
- Access Salient Vision® API: Familiarize yourself with the Salient Vision® API (Application Programming Interface). This API provides functions for accessing the sensor data, controlling the cameras, and interacting with other features of the software.
- Write Code to Acquire Data: Use the API to write code that retrieves the raw sensor data from the defined ROIs. This might involve capturing images, video streams, or other sensor readings, depending on your application.
- Store Acquired Data: Store the acquired data in a suitable format (e.g., image files, databases) for further processing and analysis.
3. Implement Measurement (extract_metrics
)
- Identify Relevant Metrics: Determine the specific measurements you need to extract from the acquired data. These could be object counts, object sizes, speeds, positions, or other relevant features depending on your application.
- Utilize Salient Vision® Tools: Explore the built-in tools and algorithms within Salient Vision® for extracting these metrics. The software might provide pre-built functions for object detection, tracking, and measurement.
- Develop Custom Measurement Functions (if needed): If the built-in tools don’t fully meet your needs, you may need to write custom code using the API to extract the specific metrics you require.
4. Implement Analysis (analyze_data
)
- Apply Analytical Algorithms: Use Salient Vision®’s built-in algorithms or develop your own custom algorithms to analyze the extracted measurements. This could involve statistical analysis, pattern recognition, trend identification, or other techniques to derive meaningful insights from the data.
- Integrate with External Tools (Optional): If necessary, integrate Salient Vision® with external data analysis tools or platforms to perform more specialized analysis or combine the data with other data sources.
5. Implement Reporting (generate_report
)
- Use Salient Vision® Reporting Features: Explore the reporting capabilities within Salient Vision® to generate reports that summarize the analysis results. This might involve creating visualizations, charts, tables, or customized reports.
- Export Report Data: Export the report data in various formats (PDF, CSV, etc.) for sharing, archiving, or further analysis.
6. Implement Evaluation and Review (evaluate_results
and review_and_adjust
)
- Define Evaluation Criteria: Establish clear criteria for evaluating the results of the SMARTER process. This could involve accuracy targets, performance benchmarks, or other relevant metrics.
- Develop Evaluation Procedures: Create procedures for assessing the results against the defined criteria. This might involve manual review, automated checks, or a combination of both.
- Implement Feedback Mechanisms: Establish mechanisms for incorporating feedback from users, stakeholders, or the system itself to continuously improve the SMARTER process. This could involve regular reviews, automated alerts, or user input forms.
Example using Python and Salient Vision® API:
Python
# ... (previous code from initial response)
def acquire_data(data_source):
# Example using Salient Vision® API
camera = SalientVision.Camera(data_source) # Connect to the camera
image = camera.capture_image() # Capture an image
return image
def extract_metrics(image):
# Example using Salient Vision®'s object detection tool
detector = SalientVision.ObjectDetector()
objects = detector.detect(image) # Detect objects in the image
measurements = []
for object in objects:
measurements.append({
"object_id": object.id,
"position": object.position,
"size": object.size
})
return measurement
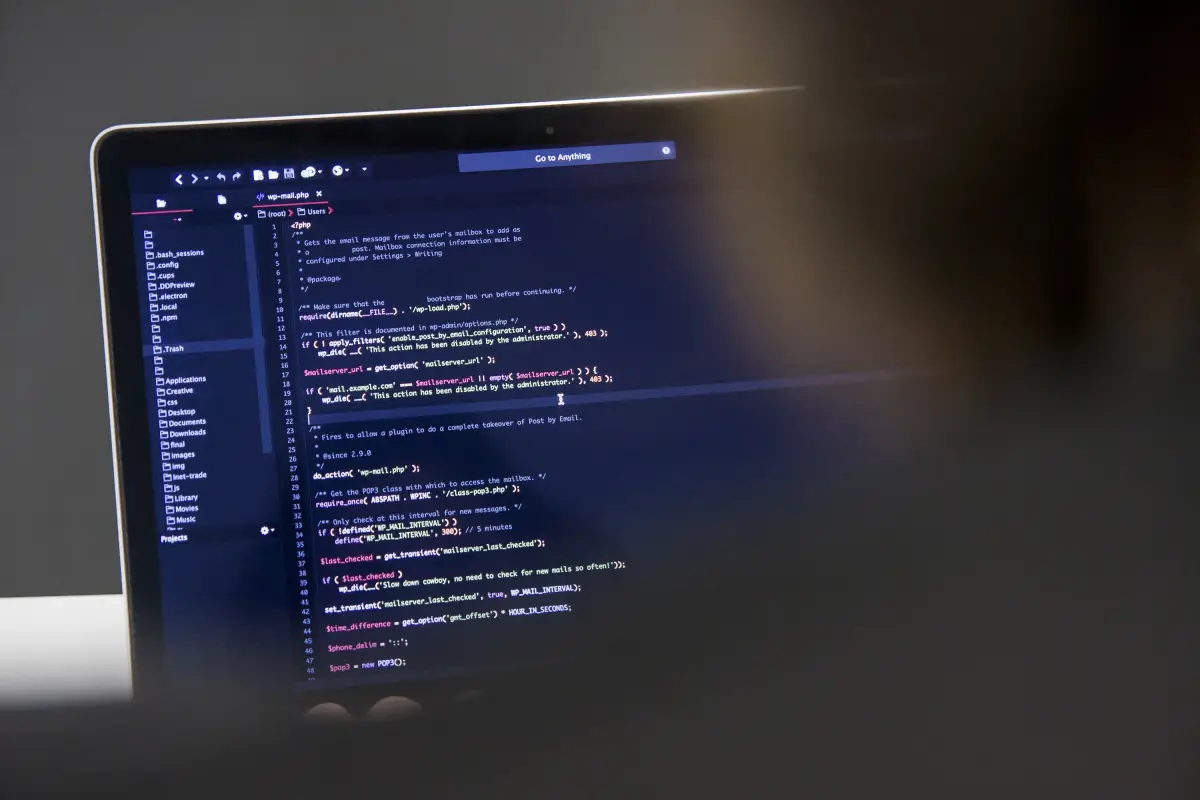